Practical Backend Developer Roadmap 2025
Prefer a video instead? Here you go: https://youtu.be/kTL0kTKt8LM
If you just master databases in depth and API design, you'll become unstoppable. 💪
🛠️ Guiding Principles
1️⃣ Frameworks come and go, fundamentals are forever. Learn the core concepts that apply across all languages and tools.
2️⃣ Practice. Practice. Practice. Reading is easy; implementing is where real learning happens.
3️⃣ Show, don’t tell. Build projects that prove your skills.
4️⃣ Avoid tutorial hell. Stick to a structured roadmap instead of jumping between random resources.
5️⃣ It's a marathon, not a sprint. Consistency matters more than speed.
6️⃣ Anyone can learn it. No CS degree required!
7️⃣ Have fun. Treat it like a game, break things, and fix them.
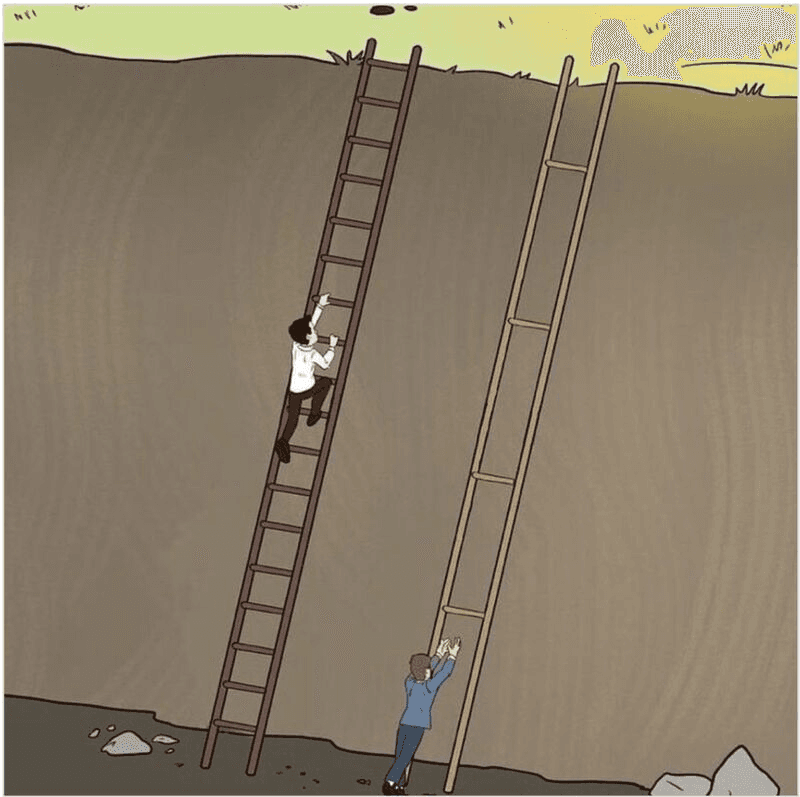
📌 Prerequisites (What You Should Know Before Starting)
✅ Comfortable with one programming language (arrays, objects, loops, functions).
✅ Can use Git to track code changes.
✅ Can navigate the command line (basic shell commands).
✅ Know how to debug errors using print statements or an IDE.
✅ Understand what an API is and how the internet works (basic HTTP).
✅ Can Google effectively when stuck.
🗺️ The Roadmap
Step 1: Mastering SQL 🔥 (Don’t skip this!)
Data Modeling in SQL
Creating, Altering, Dropping Tables
Primary & Secondary Keys
Composite Keys
Constraints:
UNIQUE
,DEFAULT
,NOT NULL
Choosing the Right Data Types
Writing Queries
Fetching data:
SELECT
,WHERE
,ORDER BY
,LIMIT
Aggregation:
COUNT
,SUM
,AVG
,MIN
,MAX
,GROUP BY
,HAVING
Filtering:
IN
,BETWEEN
,LIKE
,CASE WHEN
Insert, Update, Delete
Step 2: Database Design & Relationships
When to create a new column vs. a new table?
Relationships: One-to-One, One-to-Many, Many-to-Many
Foreign Keys & Indexing
Step 3: Working with Multiple Tables
Joins:
INNER JOIN
,LEFT JOIN
,RIGHT JOIN
,FULL JOIN
UNION vs. UNION ALL
Step 4: API Design (Backend Core!)
Understanding REST APIs
HTTP Methods (
GET
,POST
,DELETE
, etc.)HTTP Status Codes (
200
,404
,503
, etc.)
Building APIs
CRUD Operations on a Database
Using an ORM (e.g., Prisma, TypeORM, SQLAlchemy)
API Naming Best Practices
Integrating 3rd-party APIs
Calling external APIs
Handling API rate limits
Step 5: Authentication & Access Management
Authentication vs. Authorization (They are NOT the same!)
Basic Authentication (username/password)
OAuth 2.0 & Social Logins
JSON Web Tokens (JWT) & Session-based Auth
Middlewares for Access Control
Step 6: Working at Scale
Add caching to improve API response times.
Log incoming requests for debugging purposes.
Deployment Pipeline (CI/CD): Deploy online to a platform of your choice (Vercel, Netlify, Render, etc.) on pushing a commit to GitHub.
🚀 Capstone Project (Final Challenge!)
Build a feature-rich backend system that includes:
✅ A database with proper relationships
✅ A well-designed API with authentication
✅ Deployment on a real server
Other examples:
A job board where users can post/apply for jobs
A URL shortener with analytics
A to-do list with due dates and notifications
🔥 This roadmap is not about learning everything—it’s about learning what matters.
Follow it, build projects, and you’ll be ahead of 90% of backend devs out there. 🚀
Feel free to share your capstone project link in an email to swapnil@cactro.com